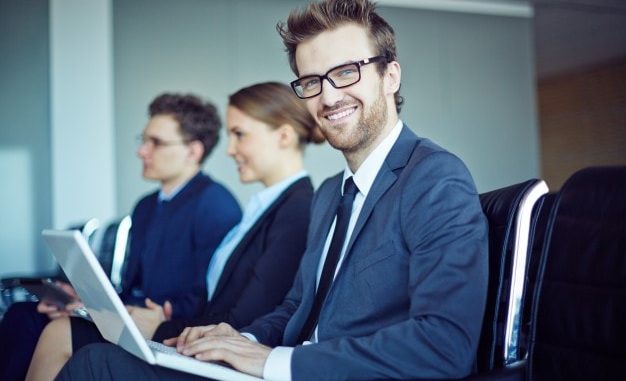
How to verify if the app is properly signed?
As you probably know, and as we have already talked about, Android always requires that all of the APKs are digitally signed. They need to be signed with the certificate even before they can actually be installed. Such a digital signature must be required by the Android’s system. And we have said, all of that has to be done before the Android system installs or runs the app. Besides that, all of the process parts are also used when verifying the identity of the owner for some future updates of the app if they may be required. Such a process can actually prevent the app from being tampered with, or even modified, in which case it could include some malicious code. We don’t want that, right?
Once the APK is signed, there is the public-key certificate that is always being attached to the APK. It uniquely associates the APK developers and also their corresponding key which is private. Let’s say that the process of building the app started. If it happens in the debug mode, then the Android SDK signs the app with a key which is specially designed for the debugging. Although, it isn’t designed for the distribution. Also, it won’t be accepted in most of the app stores (for example-Google Play Store). How can the app then be prepared for the final release? It needs to have the valid assignment which contains the release key that actually belongs to the developer.
What would be the final release build? It is a process where the app must be signed with the valid release key. If you want it to be done manually, you can easily complete such a task in the Android Studio. Just create a signing configuration and then after you have finished that, release the build type.
I would like to understand that the Android always expects some updates. If you want your app to be signed with the validate certificate, look for those that have the validity duration.
There are the two schemes of the APK signing which are available at the moment. Those are v1 scheme or JAR signing and v2 scheme called APK Signature Scheme. If you remember, and I hope that you do, we already talked about this schemes in the previous lessons. The second scheme can offer you more improved security and performance because it is built on the Android 7.0 version. But, keep in mind that the release build needs to be signed by using both schemes always.
All you have to do is to verify if the release build is signed properly-both with the scheme v1 and v2. Also, check if such a code sign certificate is containing the APK which belongs to the developer.
How to examine the content of the signing certificate? You can do it easily with jarsinger. Static analysis is recommended to use when you want to verify your APK signature.
They can be easily verified by using the apksinger tool this way:
$ apksinger verify –verbose Desktop/example.apk
Verifies
Verified using v1 scheme (JAR signing): true
Verified using v2 scheme (APK Signature Scheme v2): true
Number of signers: 1.
Also, it is a good thing to know how the APK which is signed with a Debug certificate looks like:
$ jarsinger -verify -verbose -certs example.apk
sm 11116 Fri Nov 11 12:07:48 ICT 2016 AndroidManifest.xml
X.509, CN=Android Debug, 0=Android, C=US
(certificate is valid from 3/24/16 9.18 AM to 8/10/43 9:18 AM)
(CertPath not validated: Path does not chain any of the trust authors).
When you want to identify the buffer overflows which may exist, you need to know which are the red flags and how do they look. let’s see the list:
-‘strcat’,
-‘strcpy’,
-strlcat’,
-‘strncat’,
-‘strlcpy’,
-‘snprintf’,
-sprintf and
-‘gets’.
Here, when you use the integer variables, let’s say for array index or buffering the length calculations, you need to verify that the integer types that are unsigned are used and also that the precondition tests are performed with a purpose to prevent any possibility of the integer wrap. You must know how the app never uses the unsafe strings function which could be for example-strcpy. Also, many other which starts with the same prefix-sprint, gets and all that we have already listed. ANSI C++ strings are always used if the application contains that C++ code. Those apps which are used in iOS and written in the Objective-C are always using the NSString class.
What about the dynamic analysis? Here, first things you should remember is that all of the memory corruption bugs are always easily discovered by input fuzzing. It is the automated black-box software which tests the techniques where the malformed data has been sent all the time. It will do it to check if any of the vulnerabilities may exist. They also generate the multiple instances of the structured input in some kind of the semi-corrected fashion.
Android: debuggable. This is the attribute which lays in the Application element. It determines should the app be debugged or not. It does it, of course, for the apps which are running in a user mode which is built on the Android.
When it comes to releasing build, it is important to remember that this attribute needs to be set to default, and by that we mean to ”false”.
We talked about Drozer already. How can it be used when identifying if the app itself is debuggable? It has the module app.package.attacksurface which displays the information about IPC components that are exported from the app. So, it does it, in addition, to check if the application is debuggable. But what if you want to scan for all of the debuggable apps on your mobile device? You can turn to the module app.package.debuggable.
Okay, let’s say you found a debuggable app. Now, it is very important to set the command of the execution in the context of the app. You can do it by this: find the adb shell and then execute the run-as binary.
Android Studio can help you to debug the app and also to verify it if debugging is activated for that particular application. You can also attach the jdb to the process which is running. If you have done it all successfully, the debug is activated.
I would like to say few more tips and advice about debugging. There is a tool named JADX which can also be used when identifying some interesting locations where the breakpoints should be actually inserted.
How to test the injection flaws?
We will now take a look at the list where the Android apps can expose their functionality. Those would definitely be the apps with the IPC mechanisms, like Binders, Intents, BroadcastRecievers and more. They can also show their functionality via some custom URL schemes which are usually also some parts of the intents and the user through its interface.
Although, you need to know that whatever the input is coming from this sources, it just cannot be trusted. You need to validate and sanitise it before being sure if they are safe. Such a process will ensure you that the data is the only one which is proceeded and the only one application is actually expecting. If you don’t enforce this act, the attacker may easily exploit some vulnerable functionalities to the application.
Also, the source code needs to be checked. It can be done through some custom URL schemes, user interface, and IPC mechanisms.
The Exception Handling-Testing
How can the exceptions occur? They often come up when the app gets into some non-normal or even erroneous state. It is the truth that they can be thrown, and that is possible both in the Java and C++ exceptions. This testing is all about reassuring that the app will actually be able to handle the exception and also to get the safe state. All that, and without exposing any of the sensitive information at both the UI and the logging mechanisms which are used by the application.
If you want to understand how the application handles some various types of errors, then you need to review the source code.
This lesson was a bit shorter, but don’t let that trick you! It is important as all of the other! Try to understand them as a chain…